A Complete Tutorial on Deleting a WordPress Post Using AJAX
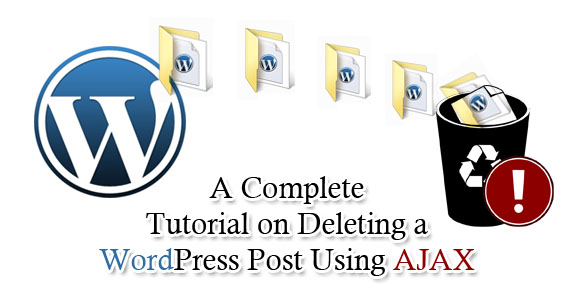
AJAX, which stands for Asynchronous JavaScript and XML, is a remarkable web technology that enables a web page to perform actions or updates dynamically without needing to reload it. A Website, that uses Ajax technology, is more interactive and responsive than a non-Ajax site. This technology not only helps developers make useful tools and better interfaces, but also lets them optimize code and minimize bandwidth usage.
As AJAX bridges the gap between client-side and server side code, it is being used by all types of web sites including WordPress. For instance, Google Docs and Google Maps utilize this technology to process the user’s request without reloading the web page. However AJAX can be used freely anywhere, but WordPress has built-in support for it. In WordPress, it is used to perform instant updates that include comment moderation and adding or deleting items from lists such as posts, categories etc. In this tutorial, we’ll show you how to delete a WordPress Post Using AJAX.
Let’s start by understanding AJAX flow.
The AJAX Flow:
To create something using AJAX, you’ll require following things:
- An action to trigger the call
- An AJAX call
- Server side code which is executed
- JavaScript code to process the results
Though all the above listed things are not required all the time, but we’ve shown you how it generally goes. Let’s go ahead to know how AJAX can be useful in deleting a WordPress post.
If you’ve a WordPress site that has a huge number of posts, you’ll be able to delete posts from the front-end. To delete a post, you’re required to click a link that uses a script to delete the post and redirects you back.
Using AJAX, you can fade the post out without reloading entire page. In this deletion process:
- The trigger is the link which we’ll click to delete the post.
- An AJAX call will contain an identifier to know which post to delete and a security nonce.
- On the server side, the data passed by the AJAX call will be used to delete the post.
- On the front-end, the post will be faded out.
The Trigger:
All you’ve to do is create a link, and then give it a class name that will be used to detect the click using JavaScript. Use the following snippet of code:
< pre class=”lang:default decode:true” >
< div < .h1 >< a href=”< ?php the_permalink() ?>”>< ?php the_title() ?>< \h1 >
< div class=”post-content” >
< ?php the_content() ? >
< /div >
< ?php if( current_user_can( ‘delete_post’ ) ) : ? >
< a href=”#” data-id=” < ?php endif ? >
< /div >
< /pre >
Here, the current_user_can() parameter is used to check whether or not the current user has permissions to delete a post and only output the link when required. Additionally, the “id” has been added as a data attribute to the link which will be clicked by user to delete the post.
In many cases, you can also add “id” to the starting post div. This way there is no need to add it as a data attribute to all links, when you’ve multiple control links. Instead, you can check the id of the common ancestor.
The “nonce” is added as the data-nonce parameter. When a user will click the link, the script will check the existence of “nonce” to ensure that the user who clicked on the link has the rights to delete the post. Later on, we’ll add a real URL instead of “#” sign, so it can also work without using JavaScript.
The Call:
In this step, an AJAX call will be performed through JavaScript to detect the link, and then it’ll send data to the server for processing. Before writing the JavaScript code, we’ll show you how to add JavaScript files to WordPress. Add the following code to the theme’s functions.php file:
< pre class=”lang:default decode:true” >
function my_frontend_script( ) {
wp_enqueue_script( ‘my_script’, get_template_directory_uri( ) .
‘/js/my_script.js’, array( ‘jquery’ ), ‘1.0.0’, true );
}
add_action( ‘wp_enqueue_scripts’, ‘my_frontend_script’ );
< /pre >
In the above code, the wp_enqueue_script() is used to tell the WordPress that you’ve added a script to the header of your theme. Keep in mind that you’ll require to use the admin_enqueue_scripts hook for the WordPress backend.
In WordPress, all AJAX calls should be routed through the admin-ajax.php file that resides in wp-admin. In JavaScript, there’s no way of doing this as everyone’s WordPress has a different location. Fortunately, there is a method through which you can pass variables to your JavaScript functions by using the function localize_script(). Add the code given below into the my_frontend_script() function, after enqueuing your script:
wp_localize_script( 'my_script', 'MyAjax', array( 'ajaxurl' => admin_url( 'admin-ajax.php' ) ) );
This function passes the “MyAjax” object to your script, so you can use the variables that it contains. Now you can write your JavaScript as shown below:
jQuery( document ).ready( function($) { $(document).on( 'click', '.delete-post', function() { var id = $(this).data('id'); var nonce = $(this).data('nonce'); var post = $(this).parents('.post:first'); $.ajax({ type: 'post', url: MyAjax.ajaxurl, data: { action: 'my_delete_post', nonce: nonce, id: id }, success: function( result ) { if( result == 'success' ) { post.fadeOut( function(){ post.remove(); }); } } }) return false; }) })
The above JavaScript code will detect whenever a user will click on the delete post link. Be sure to use the return false function at the end, so the link will not be followed. This script will remove the post ID, the security nonce and the post element from the HTML.
The parameter “action” must be passed in the above code, as the AJAX call will be performed by using the Ajax URL that we’ll define and pass to the script. Also, we’ll pass the nonce and id to the server-side script.
If the server returns “success” message to JavaScript, then the post will be faded out. Note that the success of an AJAX call doesn’t depend on the success of the deletion process. The call will still be successful, even if the item is not deleted.
On The Server:
Open the theme’s function.php file once again, and add the following script by which the server will delete the post.
add_action( 'wp_ajax_my_delete_post', 'my_delete_post' ); function my_delete_post(){ $permission = check_ajax_referer( 'my_delete_post_nonce', 'nonce', false ); if( $permission == false ) { echo 'error'; } else { wp_delete_post( $_REQUEST['id'] ); echo 'success'; } die(); }
As Hooks are created dynamically according to the action, the prefix wp_ajax_ we’ve used before the action will figure out the name of your hook. You can also prefix your action with wp_ajax_nopriv_, if you want to allow non-logged in users to execute something.
After the function is created, a security nonce is used. The check_ajax_referer() will use the name of the action, the parameter name in which it is passed and an argument to make the script die on failure.
When we perform deletion, then it’ll either return “success” or “error”. If it returns “success”, it means the user has permission to delete the post. In that case, it will be detected by our JavaScript and the post will be removed from the DOM.
If it returns “error”, when the user doesn’t have permission to delete the post, nothing will happen.
Graceful Degradation:
The most interesting thing about the AJAX workflow is that you need to do nothing additional to make it work without JavaScript. As AJAX calls pass POST or GET parameters, so all you need to do pass parameters via actual link. So modify your code for the post by adding the actual URL instead of “#” sign.
< pre class=”lang:default decode:true” >
< div < .h1 >< a href=”“>< \h1 >
< div class=”post-content” >
< ?php the_content() ? >
< /div >
< ?php if( current_user_can( ‘delete_post’ ) ) : ? >
< ?php $nonce = wp_create_nonce(‘my_delete_post_nonce’) ? >
< a href=” < ?php endif ? >
< /div >
< /pre >
Here, no major changes are made and the same nonce, action and ID are used as before. The only difference is that we’ve used $_GET variables in place of $_POST. Internally, The < i >check_ajax_referer()< /i > uses the $_REQUEST. As we’ve already used it in the < i >wp_delete_post< /i > function, there are no chances of any type of problem.